Home¶
Overview¶
startorch
is a Python library to generate synthetic time-series.
As the name suggest, startorch
relies mostly on PyTorch to generate the time series and to control
the randomness.
startorch
is built to be modular, flexible and extensible.
For example, it is easy to combine multiple core sequence generator to generate complex sequences.
The user is responsible to define the recipe to generate the time series.
Below show some generated sequences by startorch
where the values are sampled from different
distribution.
![]() |
![]() |
uniform | log-uniform |
![]() |
![]() |
sine wave | Wiener process |
Motivation¶
Collecting datasets to train Machine Learning models can be time consuming.
Another alternative is to use synthetic datasets.
startorch
provides modules to easily generate synthetic time series.
The user is responsible to define the recipe to generate the time series.
The following example shows how to generate a sequence where the values are sampled from a Normal
distribution.
from startorch.sequence import RandNormal
generator = RandNormal(mean=0.0, std=1.0)
batch = generator.generate(seq_len=128, batch_size=4)
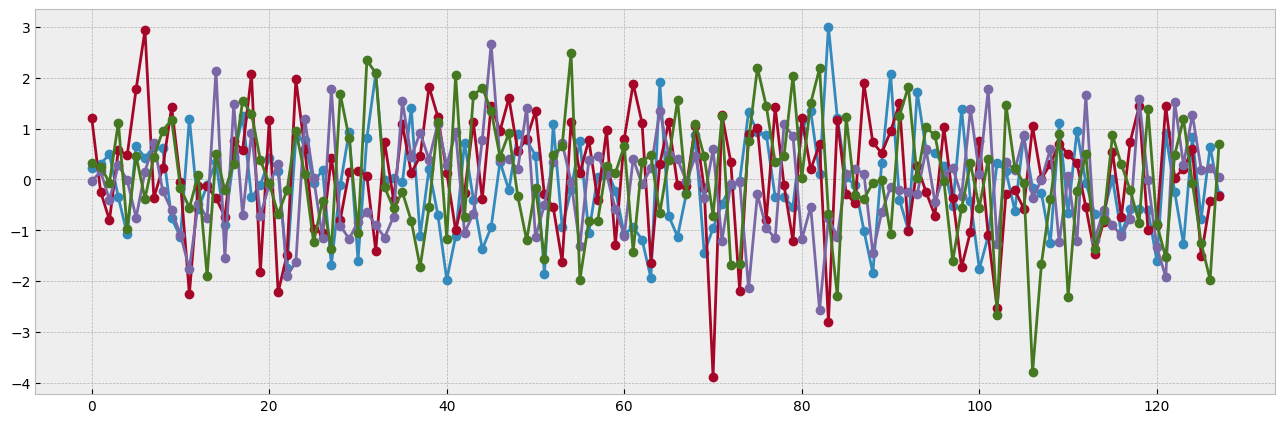
It is possible to combine multiple generators to build a more complex generator. The example below shows how to build a generator that sums multiple the output of three sine wave generators.
from startorch.sequence import (
Add,
Arange,
Constant,
RandLogUniform,
RandUniform,
SineWave,
)
generator = Add(
(
SineWave(
value=Arange(),
frequency=Constant(RandLogUniform(low=0.01, high=0.1)),
phase=Constant(RandUniform(low=-1.0, high=1.0)),
amplitude=Constant(RandLogUniform(low=0.1, high=1.0)),
),
SineWave(
value=Arange(),
frequency=Constant(RandLogUniform(low=0.01, high=0.1)),
phase=Constant(RandUniform(low=-1.0, high=1.0)),
amplitude=Constant(RandLogUniform(low=0.1, high=1.0)),
),
SineWave(
value=Arange(),
frequency=Constant(RandLogUniform(low=0.01, high=0.1)),
phase=Constant(RandUniform(low=-1.0, high=1.0)),
amplitude=Constant(RandLogUniform(low=0.1, high=1.0)),
),
)
)
batch = generator.generate(seq_len=128, batch_size=4)
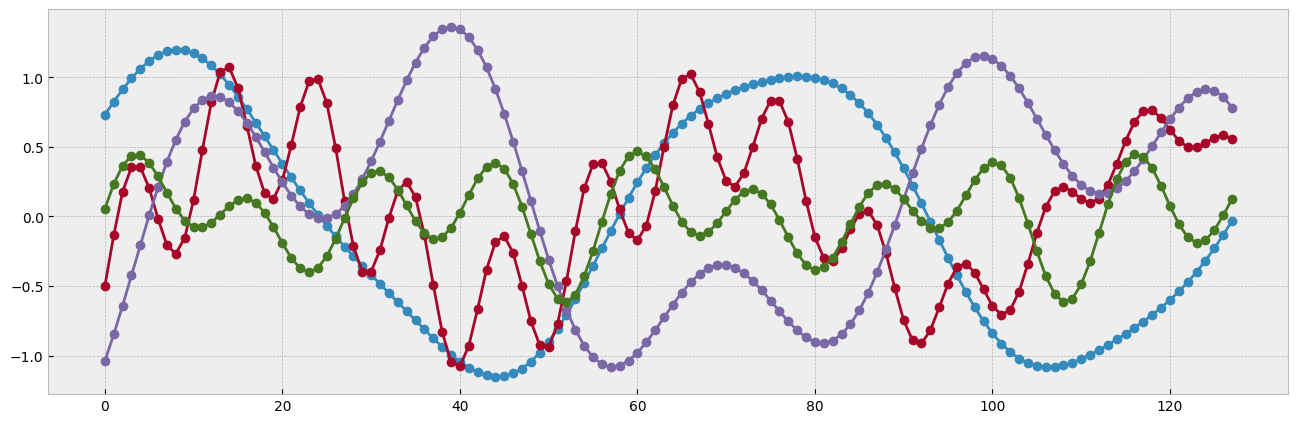
API stability¶
While
startorch
is in development stage, no API is guaranteed to be stable from one
release to the next. In fact, it is very likely that the API will change multiple times before a
stable 1.0.0 release. In practice, this means that upgrading startorch
to a new version will
possibly break any code that was using the old version of startorch
.
License¶
startorch
is licensed under BSD 3-Clause "New" or "Revised" license available
in LICENSE file.